Mobile SDKs support
One notable benefit of utilizing web technologies for the UI is its adaptability for mobile platforms like iOS and Android with minimal effort. Both mobile SDKs incorporate a web technology-based mapping layer, functioning as an interface between the Mobile and Web Player APIs. This mapping layer serves as an adapter for the Player APIs and PlayerEvents, ensuring seamless integration.
Compatibility
When employing a unified UI for both web and mobile platforms, it is crucial to verify the compatibility of new features or modifications across all utilized platforms. When integrating a newly introduced Player API or PlayerEvents into a UI component, it is essential to maintain backward compatibility and ensure compatibility with the mobile SDKs. This can be achieved by verifying the presence of a specific API or Event within the current player instance.
Not all APIs and Events are available on the Mobile SDKs
See Player communication - Backwards compatibility for details how to dynamically check if an API or PlayerEvents is available.
When developing a component exclusively for the Mobile SDKs, an API may only be accessible through one platform and unavailable on others. For instance, consider the Playlist API.
In the playlist feature, a single player instance sequentially plays various sources. This source switch is indicated through the PlaylistTransitionEvent
which only exists on mobile. To ensure the accurate display of UI metadata, such as title, description, and duration, the user interface must be designed to respond to these transition events.
However, the UI is written in TypeScript and built against the Web Player types where the PlaylistTransitionEvent
does not exist. To compensate for this, the type for the mobile unique event or API needs to be explicitly declared for the type system.
Find a complete example in mobilev3playerapi.ts.
Debugging on mobile
The easiest way to develop and debug the UI for mobile is to specify the URL to your development environment.
Chekcout the README of our UI repo how to get started with local development.
- Use the URL to your local server in the appropriate configuration
playerConfig.styleConfig.playerUiJs = URL(string: "http://your-ip:9000/js/bitmovinplayer-ui.js")!
playerConfig.styleConfig.playerUiCss = URL(string: "http://your-ip:9000/css/bitmovinplayer-ui.css")!
val viewConfig = PlayerViewConfig(
uiConfig = UiConfig.WebUi(
jsLocation = "http://your-ip:9000/js/bitmovinplayer-ui.js",
cssLocation = "http://your-ip:9000/css/bitmovinplayer-ui.css"
)
)
Make sure that your devices are in the same network.
- Enable Web View debugging
iOS:
- Make sure to enable the WebView inspection on your phone inside the system settings at Settings → Safari → Advanced -> Web Inspector.
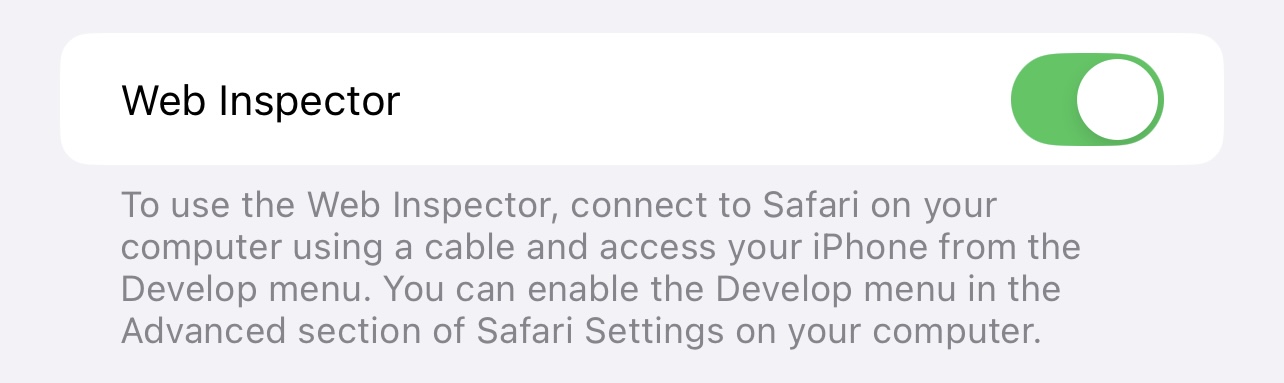
- And enable web view inspecting in our SDK through the
StyleConfig
.
let bitmovinUserInterfaceConfig = BitmovinUserInterfaceConfig()
bitmovinUserInterfaceConfig.enableWebViewInspecting = true
styleConfig.userInterfaceConfig = bitmovinUserInterfaceConfig
- After enabling WebView inspection, you can use Safari to debug the UI. See Apple Documentation - Inspecting iOS for details.
Android:
Enable WebView debugging statically using WebView.setWebContentsDebuggingEnabled
.
WebView.setWebContentsDebuggingEnabled(true);
See Remote debugging WebViews for details.
- Once you can access the WebView within your Application, you can use the browser console features as usual.
Updated about 1 month ago